Frequently Asked
Questions on GTCS1Lib
General Code/Logic
Questions
- Q: My EchoToStatus messages are not showing up why?
A: You probably did not
include the Font in your Content project.
Do this:
Look at the “UsefuleFiles” folder
from any of the week-1 examples (e.g., 1.Speed). You will see the “Resources”
folder. Make sure:
·
Copy the entire Folder into your project’s
Content folder, so in your project’s “Content” folder you will see:
o Content.contentproj
o Resources
(which has three sub-folders:
§ Textures
– can be empty
§ Audio
– can be empty
§ Fonts
– Arial.spritefont.
See
if your messages show up now?
- Q: Audio won't stop: Here
is my code in UpdateWorld():
if (mBall.CenterX < 0f){
PlayACue("BelowZero");
}
How come my audio cue plays continuously?
A: Because you did not update the
mBall.CenterX position after you play the audio cue. Your logic here says, if
mBall.CenterX is less than 0, then play the audio cue. Whenever this condition
is true, the audio cue plays. Here you need to figure out when you want
to play the cue:
- A Condition: whenever
the mBall.CenterX is less than 0 or
- An Event: the
"first time" when mBall.CenterX becomes less than 0.
Figure
out what you want to do and code accordingly. To support the "event",
one way may be:
if
(mBall.CenterX < 0f) {
PlayACue("BelowZero");
mBall.RemoveFromAutoDrawSet(); // Make the ball
disappear
mBall.CenterX = 1f;
// ... later on your logic should/may decide to
// add the
ball back to the auto draw set
}
- Q: Condition not becoming true: Here is my code in
UpdateWorld():
if
(mBall.CenterX == 4f){
PlayACue("TouchesFour");
}
I can see my ball fly across the X=4f line, but the audio cue won't play, why?
A: Do not test for floating point
equality! The chance of a general floating number equal to another one is close
to zero! In general, you can only test for floating point equality when
you have specifically set a variable to a well known constant (e.g., X==0f).
Otherwise, you should test for inequality. In this case, you can test for if
your CenterX is greater than 4f.
Bugs/Features with GTCS1Lib:
XNACS1Rectangle.SetEndPoints(aPos, bPos, Width)
This function has a nasty bug: it re-sets all attributes (shouldTravel, color,
emitter, visible, … everything!) for the primitive!! Avoid using this function
for now!!
- XNACS1Primitive: bug or feature
XNACS1Rectangle.Collided(aPrimitive,
collisionPosition)
CollisionPosition is supposed to be an approximated collision
position. Sometimes,
for some reason, collisionPosition seem to always return (0,0). We should avoid
using this function, unless you can verify it works otherwise. If your primitives are textured,
you should use:
XNACS1Primitive.TextureCollided(aPrimitive,
collisionPosition)
Make sure the
texture on aPrimitive
is of higher
resolution. If you don’t understand what is being said. ASK this in class!!
- XNACS1Primitive.Sprite: bug and/or feature
XNACS1Rectangle.SetTextureSpriteSheet()
Sometimes subsequent tiles in a spritesheet “bleed” into their neighbors. This
can be observed when you notice a very thin line at the boundary of your sprite
object. In this worst case, this line “blinks” (sometimes showing, sometimes
does not show). This is a problem because we support floating point coordinate
system while the underlying XNA drawing is based on integer. Here is an
example, two 32x32 tiles from a spritesheet. The Red line is located at
pixel-32 and should only appear when we draw the right-tile.
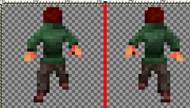
Drawing
the above left-tile on a 183x183 pixel rectangle looks like:
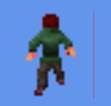
Notice
the thin “red” line to the right of the rectangle. This is “bleeded” over from
the right-tile. Unfortunately, this problem is deep in XNA and we do not have a
solution to this. The simplest workaround for this problem is to include at
least one row/column of transparent pixel between each tile in the spritesheet.
In this example, if we replace the red-line by transparent line, the problem
can be avoided. [Credit, this problem was identified and analyzed by Jonathan
Lynn in May 2011. The above example is provided by Jonathan.]
- XNACS1Base: feature
Avoid accessing this class before it has a chance of being initialized.
For example, if you try to instantiate any instance variables before the class
constructor:
public class ClassExample : XNACS1Base
{
float
a = World.WorldDimension.X;
}
The
above will crash because the World-class (XNACS1Base.World) is not initialized.
·
Q:
How can I support multiple game pad controllers?
A: By pass the XNACS1 library by (in
UpdateWorld()):
Microsoft.Xna.Framework.Input.GamePad.GetState(PlayerIndex.Two).ThumbSticks.Left.X
...
Watch
out about button-event vs button-state-poll differences! (Please refer to this FAQ question).
- Q: How can I change the font?
A: Sorry, you cannot change the fonts to
Top/Bottom status (these are meant to be error/debugging). For Primitive
labels, create your own SpriteFont, store into the Resources/Fonts folder,
and use the file name as the font name for
Primitive.LabelFont
Setting this variable to null results in default font being used.
- Q: Particle Emitter: How
to remove one properly?
A: When you want to remove an emitter (or simply want to
replace one), remember, the library is keeping track of your emitter (and
all the particles emitted by that emitter), so, you need to tell the
library you are removing the emitter, here is how: e.g., I have a mTail
emitter in my class, and I want to allocate a new one …
if (null != mTail) {
mTail.RemoveFromAutoDrawSet(); // tells the library to release the reference
mTail.RemoveEmitterFromUpdateSet();
mTail = null;
}
mTail = new XNACS1ParticleEmitter.TailEmitter(Vector2.Zero … )
- Q: When I try to run any of the XNACS1Lib programs, it
would crash with complains of HiDef video card not supported. What can I
do?
A: Thanks to Jason Weaver, for
finding this solution, take a look at:
http://gamedev.stackexchange.com/questions/4262/error-on-new-xna-4-0-game-project-no-suitable-graphics-card-found
- Q: I cannot change the color of my Circle/Rectangle
primitive, what is going on?
A: Set .Texture attribute
to "null" instead of """" (set to null instead of empty string).
- Q: How can I change the font colors of the top/bottom
status echo?
A: Check out the functions:
XNACS1Base.SetTopEchoColor();
XNACS1Base.SetBottomEchoColor();
- Q: How can I pause my game?
A: Check out:
XNACS1Base.World.Paused;
- Q: How can I resize the
application window?
A:
SetAppWindowPixelDimension(fullScreen, widthInPixel, heightInPixel); Because,
you often need to redefine world bound after the application window size
change. It is probably a good idea to call this function right at the
beginning of InitializeWorld();
- Q: On the phone, how can I
react to the device physical rotation by changing the orientation of my
application?
A:
SetPhoneOrientation(lockOrientation, DisplayOrientation), this function is a no-op when your app is not run on a
phone.
- Q: Background audio: How
to stop it?
A: PlayBackgroundAudio(null, 0f);
- Q: Background audio: What are the formats supported?
A: You can drag/drop “.wav”
files in the Resources/Audio folder in the Content project. For .mp3
files, please follow this procedure
(from David Schmieder).
- Q: Accessing to user
input and library function: how
do can I get access to user input and XNACS1Lib functions from any file:
A: From any source
file, you must include the XNACS1Lib by:
using XNACS1Lib;
And then, you
can access to user input by typing the full name to GamePad, e.g., to check for
A button click, you can:
XNACS1Base.GamePad.ButtonAClicked();
To call other
library functions, e.g., to clamp to WorldBound:
XNACS1Base.World.ClampAtWorldBound(yourObject);
- Q:
Button event vs. Button State: How can I poll a button’s current state (pressed or not pressed):
A: To test for button
pressed events, you do:
if (GamePad.ButtonAClicked()) {
// Button-A Clicked event has occured ...
To poll for
current state of button A, you can do:
if (GamePad.Buttons.A
== ButtonState.Pressed)
// currently Button-A is depressed
Problems relating
to XBOX 360:
- Q: After I signed up for my XNA Creators Club Silver,
when I try to launch "XNA Studio Connect", I receive the
error messsage: "Error 5: Premium Membership required. One or more
of the accounts does not have this ...".
A: You may have to wait a while
(like up to 4 hours) and try again! Refer to the discussion here: http://forums.xna.com/forums/t/24812.aspx
apparently it can take a while for the different servers to synch with
each other.
- Q: Can I
re-format Xbox 360 hard drive?
A: YES! Whenever you think
necessary, please do! Here is how: http://support.microsoft.com/kb/906502
- Q: Problem downloading XNA Game Studio Connect, with
error message saying: “This account is not permitted to download
content with this rating”
A: http://support.microsoft.com/kb/959240
Problems relating
to Windows Phone 7:
- Q: When I try to deploy my project from VS2010, I
received: "Zune software is not launched …"
A: Well, the Zune software is not
launched! WP7 communicates with your machine via the Zune software.
Download/Install/Launch the Zune! Your IDE can load apps to the phone only
when Zune is running! You need to plug in your phone, configure (easy)
your Zune to recognize the phone.
- Q: I have Zune running and configured it such that Zune
knows about my phone. When I try to deploy, I received: "Application
launch failed. Ensure that the device screen is unlocked and device is
developer unlocked …"
A: A few things can go wrong here:
- If you are not
registered as a WP7 developer, you need to. Follow the instruction here: this
guide to register with the vendor.
- If you are already an
“identify and verified” developer, then did you run the “Windows Phone Developer Registration”
(under: All Programs->Windows
Phone Developer Tools), you need to unlock your phone with the email
you used to register as a developer.
- Finally, well, before
you deploy, you have to make sure the phone is not in power-saving or
screen-lock mode!
- Q: I have been debugging with WP7 device no trouble, and
then today when I try to deploy I received: "Unable to install
application. The maximum number of developer applications on this phone
has been reached …"
A: Well, as far as I can tell, each
phone will only allow two app’s to be deployed. Kind of … not nice. But, I
have been leaving with this. You will need to uninstall one of your app
before you can deploy more. To uninstall, on the phone, select by
press-and-hold, you will see the uninstall menu.
- Q: When I compile my WP7 project, I got this error
message: "Error 1 Unable to determine the entry point. Assembly
‘..\bin\Windows Phone\Release\mp1Example_WP7.dll' has no visible types
deriving from Microsoft.Xna.Framework.Game. mp1Solution_WP7"
A: You forget to include: public in your class definition. As in
o class MyMP1 : XNACS1Base
and forget to make your class public as it should be:
o public class MyMP1 : XNACS1Base